Rust Developer Interview Questions Guide 2025: Your Complete Blockchain Rust Developer Handbook
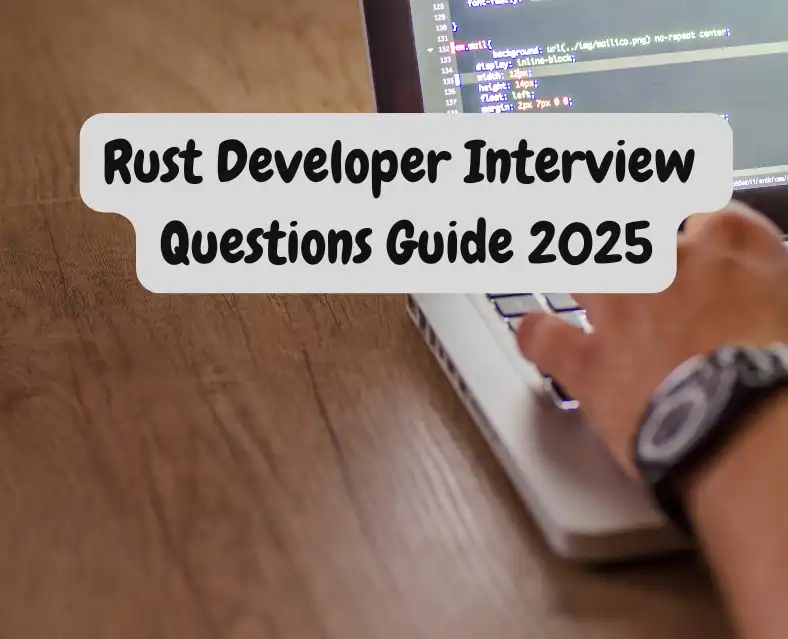
TL;DR
Why Rust? Rust is the go-to language for blockchain development due to its memory safety, performance, and concurrency features.
Market Demand: Rust adoption grew by 20% in 2024, with blockchain accounting for 35% of its use cases.
Key Skills: Master Rust programming (ownership, traits, lifetimes) and blockchain concepts (consensus, smart contracts, cryptography).
Interview Prep: 30+ sample questions with answers, including Rust programming, blockchain concepts, and practical coding tasks.
Salary Insights: Entry-level Rust developers earn 80K–100K/year in the USA, with senior roles paying up to $200K/year.
Resources: Rustlings, Cryptozombies, LeetCode, and books like “Programming Rust” are essential for preparation.
Introduction
Rust has become a cornerstone of blockchain development, offering unmatched performance, memory safety, and concurrency. Its adoption by major platforms like Polkadot, Solana, and NEAR Protocol underscores its importance in building secure and scalable blockchain systems. As decentralized technologies like DeFi, NFTs, and Web3 gaming gain traction, the demand for Rust blockchain developers is skyrocketing.
This guide is your ultimate resource to prepare for a Rust developer interview, covering everything from technical concepts to salary insights and practical coding tasks. Whether you’re a beginner or an experienced developer, this guide will help you stand out in the competitive blockchain job market.
Market Forecast for Rust Blockchain Developers
Why Rust is the Future of Blockchain Development
Rust’s unique features, such as zero-cost abstractions and a robust ownership model, make it ideal for blockchain systems. In 2024, Rust adoption grew by 20% year-over-year, with blockchain accounting for 35% of its use cases. This growth is driven by Rust’s ability to handle complex, high-performance systems securely.
Blockchain Developer Market Growth
The global blockchain developer market is projected to reach $18 billion by 2026 (source: Statista). This expansion is fueled by the rise of DeFi, NFTs, and Web3 gaming, all of which rely heavily on Rust-based platforms.
Hiring Trends
Companies like Parity, Solana, and NEAR Protocol are hiring Rust developers at a record pace, offering competitive salaries and benefits. The future looks bright for Rust blockchain developers, with demand expected to grow as decentralized technologies gain mainstream adoption.
Key Topics to Prepare for Rust Interview
Rust Programming
Ownership, Borrowing, and Lifetimes: Understand how Rust manages memory and prevents common errors like null pointer dereferencing.
Traits and Generics: Learn how to write reusable and type-safe code.
Memory Safety: Explore Rust’s guarantees against data races and memory leaks.
Blockchain Concepts
Consensus Mechanisms: Study Proof of Work (PoW), Proof of Stake (PoS), and other consensus algorithms.
Smart Contract Deployment: Learn how to write, test, and deploy smart contracts on platforms like Solana and NEAR Protocol.
Cryptography Basics: Understand cryptographic hashing, digital signatures, and public-key cryptography.
Advanced Development
Writing and Deploying Smart Contracts: Gain hands-on experience with Rust-based blockchain platforms.
Cross-Chain Communication: Explore techniques for interoperability between different blockchain networks.
Rust Developer Interview Questions & Answers
1. Explain Rust’s ownership model with an example.
Rust’s ownership model ensures memory safety without needing a garbage collector. It follows three key rules: each value has a single owner, ownership can be transferred but not shared, and values are dropped when they go out of scope. This prevents issues like dangling pointers and memory leaks, making Rust highly reliable for system-level programming and blockchain applications.
2. How do you implement generics in Rust?
Generics in Rust allow functions, structs, and enums to work with multiple data types while maintaining type safety. Instead of writing multiple versions of the same code for different types, generics enable reusable and efficient implementations. They are particularly useful for data structures and algorithms that need to handle various types without sacrificing performance.
3. What are Rust macros, and when should you use them?
Rust macros are powerful compile-time tools that enable metaprogramming, allowing developers to write code that generates other code. Unlike functions, macros operate before compilation, making them useful for repetitive tasks, debugging, and implementing domain-specific languages. They are commonly used for logging, testing, and performance optimizations in Rust projects.
4. Explain how Rust ensures thread safety.
Rust enforces thread safety through its ownership system, borrowing rules, and built-in concurrency primitives. The Send and Sync traits ensure that data is safely shared or transferred across threads without data races. By preventing multiple mutable references to the same data in different threads, Rust eliminates common concurrency bugs found in other programming languages.
5. What’s the difference between Box<T>, Rc<T>, and Arc<T>?
These are smart pointers used for different memory management strategies. Box<T> provides heap allocation with a single owner, Rc<T> (Reference Counted) allows multiple ownership in single-threaded programs, and Arc<T> (Atomic Reference Counted) is a thread-safe version of Rc<T> used in concurrent applications. Choosing the right pointer ensures efficient memory usage and safe data handling.
6. How do you handle errors in Rust?
Rust provides a robust error-handling system using Result<T, E> for recoverable errors and panic!() for unrecoverable ones. The Result type encourages explicit error handling, ensuring developers address potential failures instead of ignoring them. This makes Rust a reliable choice for applications where stability and security are critical, such as blockchain development.
7. What are lifetimes, and why are they important in Rust?
Lifetimes prevent dangling references by ensuring that references remain valid for as long as they are needed. The Rust compiler enforces lifetime rules to prevent memory access issues, eliminating common bugs like use-after-free. This feature is particularly useful in complex data structures and ensures memory safety without requiring a garbage collector.
8. Demonstrate the use of traits in Rust.
Traits in Rust define shared behavior, similar to interfaces in other languages. They allow multiple types to implement the same functionality without code duplication. Traits enable polymorphism and abstraction, making them essential for designing scalable and modular applications, especially in Rust-based blockchain projects.
9. How does async programming work in Rust?
Rust’s async programming model enables non-blocking operations, allowing applications to handle multiple tasks efficiently. The async and await keywords work with asynchronous runtimes like tokio and async-std, making Rust well-suited for high-performance networking and blockchain applications. This approach improves scalability and reduces latency in concurrent tasks.
10. What are the key differences between Rust and C++ for system-level programming?
Rust eliminates many of the pitfalls of C++ by enforcing memory safety at compile time without requiring manual memory management. Unlike C++, Rust prevents null pointer dereferencing, data races, and buffer overflows through strict ownership rules. This makes Rust a safer and more modern alternative for system-level programming, particularly in security-critical applications like blockchain and cryptographic systems.
Blockchain Concepts for Rust Developers
1. What is blockchain consensus, and how would you implement Proof of Stake using Rust?
Blockchain consensus ensures all network participants agree on the state of the ledger. Proof of Stake (PoS) selects validators based on their staked assets rather than computational power, making it energy-efficient. Rust's memory safety and concurrency make it ideal for implementing PoS, ensuring secure validator selection and efficient state transitions.
2. How does Rust improve the security of smart contracts on blockchain platforms like Solana?
Rust prevents memory leaks and buffer overflows, which are common vulnerabilities in smart contracts. Solana uses Rust to enforce strict memory management, reducing attack vectors like reentrancy and integer overflows. Its ownership model ensures safe concurrency, preventing unintended state modifications.
3. Explain the concept of Merkle Trees and their implementation in Rust for blockchain applications.
A Merkle Tree is a hierarchical hash structure that ensures data integrity in a blockchain. It enables efficient verification of large datasets by storing only the root hash instead of all transactions. Rust’s type safety and cryptographic libraries make it an excellent choice for implementing Merkle Trees, ensuring secure and high-performance data verification.
4. What is the role of cryptographic hash functions in blockchain, and how do you use SHA-256 in Rust?
Cryptographic hash functions like SHA-256 secure blockchain data by creating fixed-size, unique hashes for transactions. They ensure immutability and prevent tampering by linking blocks through hash pointers. Rust provides optimized cryptographic crates like sha2, ensuring high-speed, secure hashing with minimal resource usage.
5. How does Rust's performance optimization benefit blockchain scalability and transaction throughput?
Rust’s zero-cost abstractions and low-level control over memory improve blockchain scalability. Unlike garbage-collected languages, Rust avoids runtime overhead, leading to faster transaction validation and lower latency. This makes it ideal for high-performance blockchains like Solana and NEAR, which require efficient parallel processing.
6. Describe how a blockchain Rust developer integrates cryptographic algorithms into decentralized applications (dApps).
A Rust developer leverages cryptographic libraries like ring and ed25519-dalek for digital signatures, encryption, and hashing. These algorithms secure dApps by verifying identities and ensuring data integrity. Rust’s strict type system prevents cryptographic vulnerabilities, making dApps resistant to exploits.
7. What is a UTXO model in blockchain, and how can you represent it in Rust?
The UTXO (Unspent Transaction Output) model tracks individual transaction outputs, ensuring double-spend prevention. Unlike account-based models, UTXOs allow parallel processing, improving scalability. Rust’s ownership model naturally aligns with UTXO handling, preventing state inconsistencies and optimizing transaction validation.
8. How would you implement cross-chain interoperability in a Rust-based blockchain project?
Cross-chain interoperability enables assets and data transfer between blockchains. Rust developers use cryptographic proofs, light clients, and bridges to verify cross-chain transactions securely. With its memory safety and concurrency control, Rust ensures secure message passing and state synchronization between networks.
9. Why is Rust preferred over other programming languages like Python or JavaScript for blockchain development?
Rust offers memory safety, high performance, and concurrency without garbage collection, making it ideal for blockchain. Unlike Python or JavaScript, which rely on runtime checks, Rust enforces compile-time safety, preventing vulnerabilities. This makes it a top choice for secure and scalable blockchain platforms.
10. What are some real-world examples of blockchain platforms built with Rust, and how do they utilize its features?
Solana, NEAR Protocol, and Polkadot use Rust for their core blockchain logic. Solana leverages Rust for high-performance smart contracts, while NEAR uses it for WebAssembly (Wasm)-based execution. Rust’s efficiency and safety make these platforms fast, scalable, and resistant to security breaches.
Top Blockchain Rust Developer Interview Questions with Coding Tasks
1. Question: Explain the ownership model in Rust and how it ensures memory safety.
Coding Task:
Write a Rust program that demonstrates the ownership model, including examples of moving, borrowing, and mutable borrowing.
2. Question: How does Rust handle concurrency, and why is it important in blockchain development?
Coding Task:
Implement a simple multi-threaded Rust program where two threads increment a shared counter using Arc
and Mutex
.
3. Question: What are smart contracts, and how would you implement one in Rust?
Coding Task:
Write a basic smart contract in Rust using the ink!
framework that allows users to store and retrieve a value.
4. Question: Explain the concept of consensus algorithms in blockchain. Can you implement a simple Proof of Work (PoW) algorithm in Rust?
Coding Task:
Implement a basic Proof of Work algorithm in Rust that finds a nonce for a given block hash to meet a specific difficulty target.
5. Question: How would you handle errors in Rust, and why is it important in blockchain development?
Coding Task:
Write a Rust function that parses a JSON string into a custom struct and handles potential errors gracefully using the Result
type.
6. Question: What is a Merkle Tree, and how is it used in blockchain?
Coding Task:
Implement a Merkle Tree in Rust and write a function to verify the inclusion of a transaction in the tree.
7. Question: How do you optimize Rust code for performance in a blockchain context?
Coding Task:
Write a Rust function to compute the SHA-256 hash of a block header and optimize it for performance using cargo bench
.
8. Question: What are cryptographic signatures, and how are they used in blockchain?
Coding Task:
Implement a Rust program that generates a public-private key pair, signs a message, and verifies the signature using the ring
or ed25519-dalek
crate.
9. Question: How would you implement a simple blockchain in Rust?
Coding Task:
Write a basic blockchain in Rust that includes blocks with a previous hash, timestamp, and data. Implement a function to validate the chain.
10. Question: What is the role of WebAssembly (Wasm) in blockchain, and how would you compile Rust to Wasm?
Coding Task:
Write a simple Rust function (e.g., a calculator) and compile it to WebAssembly using wasm-pack
. Demonstrate how to interact with it in a browser or Node.js environment.
Blockchain Rust Developer Salaries by Experience and Region
Salaries for Rust developers vary based on experience and location. Companies seek Rust developers for roles in blockchain, cybersecurity, cloud computing, and embedded systems. Data from Glassdoor, LinkedIn Salary Insights, and PayScale show a strong demand for Rust expertise, leading to competitive salaries worldwide.
Entry-Level Rust Developer Salaries (0–2 years)
Entry-level Rust developers often work on system integrations, open-source projects, and blockchain applications. Many start their careers at fintech firms, startups, or companies integrating Rust into their existing tech stacks. Typical salaries include:
USA: $80,000–$100,000 per year
Europe: €50,000–€70,000 per year
India: ₹12–18 LPA
While salaries at this stage depend on industry and project complexity, Rust developers tend to earn more than their counterparts working with less specialized languages.
Mid-Level Rust Developer Salaries (3–5 years)
Mid-level Rust developers take on advanced responsibilities, such as optimizing blockchain protocols, developing high-performance web applications, and working with embedded systems. Their salaries reflect their growing expertise:
USA: $110,000–$140,000 per year
Europe: €80,000–€100,000 per year
India: ₹20–35 LPA
At this stage, developers may specialize in smart contract development, cryptographic security, or parallel computing. Companies in decentralized finance (DeFi), cloud computing, and AI-driven applications actively seek Rust developers with this level of experience.
Senior Rust Developer Salaries (5+ years)
Senior Rust developers work on complex system architectures, security enhancements, and large-scale distributed applications. These professionals often hold leadership roles, managing teams and overseeing critical infrastructure projects. Their salaries reflect their advanced skill set:
USA: $150,000–$200,000 per year
Europe: €110,000–€140,000 per year
India: ₹40+ LPA
Senior Rust developers are highly sought after in cybersecurity, blockchain infrastructure, and AI-based platforms. Their ability to write secure, high-performance code makes them valuable to companies handling sensitive data and large-scale operations.
Freelance Rates for Rust Developers by Experience and Region
Rust developers charge different rates based on experience and location. Here is a clear breakdown:
United States
Entry-Level: $33 - $47 per hour
Mid-Level: $48 - $74 per hour
Expert-Level: $57 - $82 per hour
Asia & Pacific
Entry-Level: $18 - $24 per hour
Mid-Level: $24 - $35 per hour
Expert-Level: $30 - $42 per hour
Eastern Europe
Entry-Level: $25 - $42 per hour
Mid-Level: $30 - $56 per hour
Expert-Level: $45 - $70 per hour
Western Europe
Entry-Level: $20 - $27 per hour
Mid-Level: $20 - $43 per hour
Expert-Level: $30 - $40 per hour
These rates depend on demand, cost of living, and project complexity. Developers in high-demand regions charge more. Developers in regions with lower costs may offer competitive pricing. Choose a developer based on project needs and budget.
(Source: Optymize.io)
Rust Developers: Job Market Insights and Demand Trends
The demand for Rust developers is increasing as industries recognize Rust’s benefits in security, performance, and concurrency. Companies like Parity Technologies, Solana Labs, and AWS actively hire Rust developers for projects in blockchain consensus mechanisms, cloud computing, and decentralized applications.
According to LinkedIn Salary Insights and PayScale, Rust developers are among the highest-paid professionals in software development. The rise of Web3, cloud-native applications, and performance-critical systems has contributed to this demand. Developers with experience in Rust, along with knowledge of cryptography and distributed computing, can expect higher salaries and job stability in the coming years.
Rust Developers: Interview Preparation Tips and Resources
Becoming a proficient Rust developer, especially in blockchain, requires structured learning and hands-on practice. Here are some essential resources to help you prepare:
Courses
Rustlings – A highly recommended interactive course that helps you understand Rust’s syntax and core concepts through exercises.
Codecademy Rust – A beginner-friendly course that covers Rust programming fundamentals.
Cryptozombies (Solana Edition) – A blockchain-specific course that teaches Rust through smart contract development on Solana.
Books
Programming Rust – A comprehensive book that covers Rust from the basics to advanced concepts like ownership, lifetimes, and concurrency.
Blockchain Basics – Useful for understanding blockchain fundamentals if you’re transitioning from traditional software development.
Practice Platforms
LeetCode & HackerRank – Great for solving coding problems and improving problem-solving skills.
Open-Source Contributions – Platforms like Substrate provide real-world experience by contributing to blockchain-based Rust projects.
Mock Interviews
Pramp &Interviewing.io – Offer peer-to-peer mock interviews to help you practice real-world interview questions.
FAQs
Why is Rust preferred for blockchain development?
Rust is favored due to its memory safety, zero-cost abstractions, and concurrency model, making it ideal for secure and high-performance blockchain applications.
How much time does it take to become proficient in Rust for blockchain?
For developers with prior experience in C++ or Go, 3-6 months of consistent learning is sufficient. For beginners, 6-12 months may be needed.
What is the average hiring process duration for Rust blockchain developers?
Hiring can take 4-8 weeks, depending on the company and position. Expect multiple rounds, including coding assessments, system design, and blockchain-specific problem-solving.
Conclusion
The demand for Rust blockchain developers is growing, making it a valuable skill in Web3 and DeFi projects. Mastering Rust takes consistent effort, but with the right resources and practice, you can stand out in the job market. Keep honing your skills, contribute to open-source projects, and leverage platforms like artofblockchain.club for career insights and networking.